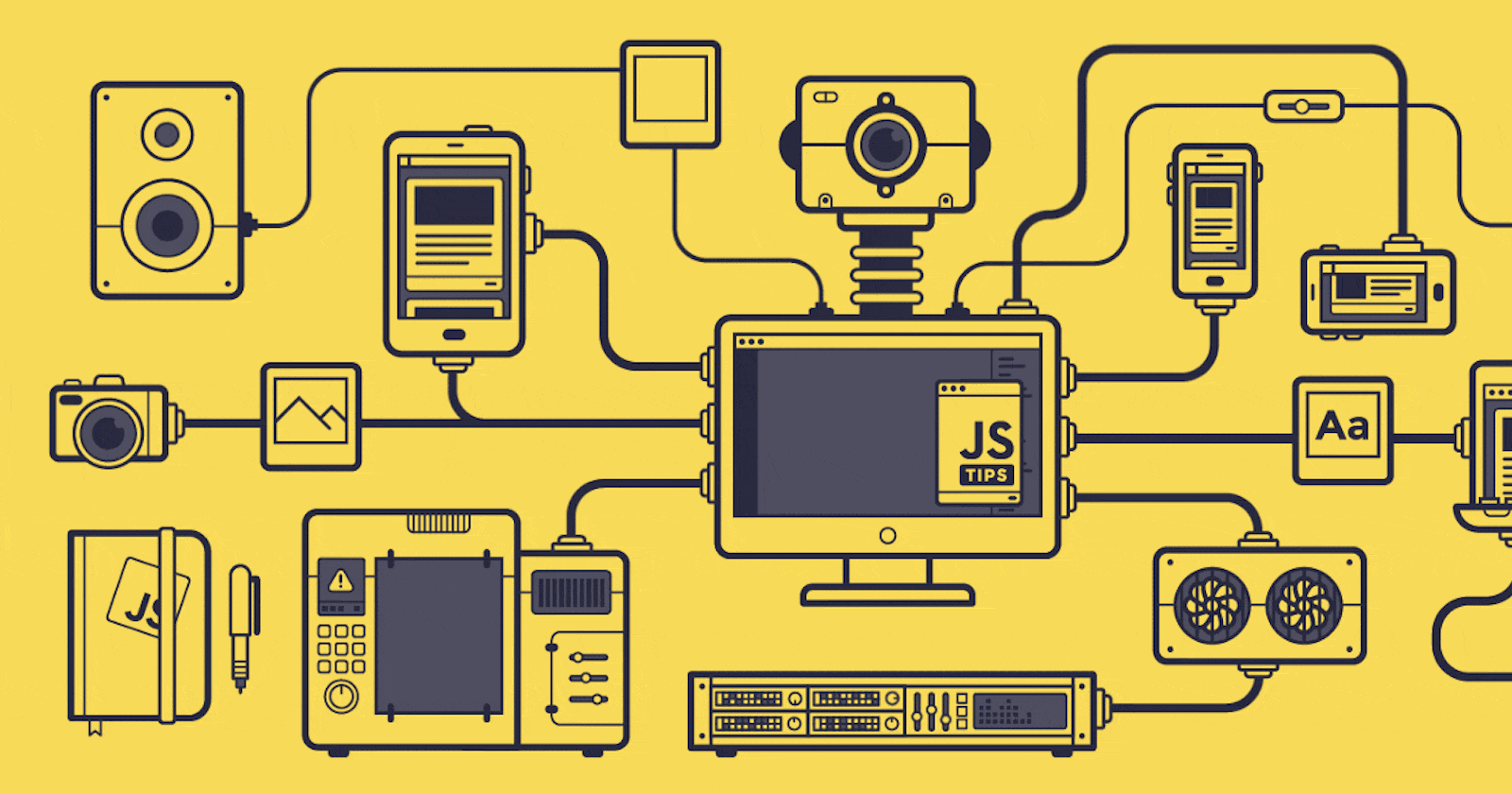
8 Must known JavaScript tricks for web developer
Grasp 8 useful JavaScript tricks that will make your web developer life easier
PermalinkJavascript
Javascript is a programming language which is mostly used to provide functionality to our website and provide a brain to our dump website that makes it wise. It can also be used in server with the help of node. Node comes up with a npm package manager which contains countless package. Node also use v8 engine which JIT (Just In Time) compilation of native code to machine code. V8 engine is the same engine which is used by Chrome for compilation of javascript native code.
Permalink1) Optional Chaining (?)
This feature allows you to read a property from object, without checking if object exists. This trick is very helpful for getting deeply nested properties from object without checking validity of each step in the chain.
user.data?.id;
user.data?.name;
user.data?.addressList?.[0];
user.greet?.();
Here ? says to javascript whether the left side value is null or not if it is not null try to fetch the value beyond ?. else just stop here. Just put it when you are not sure whether property exist or not.
Permalink2) Nullish Coalescing (Combining) (??)
It is a logical operator, that will return the right-hand side operand, only when it's left-hand side operand is either null or undefined. It is extremely useful, when we want to provide a default value when the value expected is nullish.
function getUserId(user){
return user?.name ?? "Anonymous";
}
Here ?? says to javascript whether value on left hand side is null or not. If the value is null, then store the value beyond ?? to right hand side.
Permalink3) Readable numbers
Sometimes we are not able to read the numbers properly but with the help of this property in javascript we can increase our readability of numbers.Javascript allow you to put this _ between numbers. Useit to visually divide thousands (000s).You can do all computations with these numbers and this trick also works in Python.
const normalNumber=1000000000;
const readableNumber=1000_000_000;
Permalink4) Getting unique values from Array
It is very useful trick when we get in situation to get unique values from an array. We can use a Set to achieve this objective.
const array=[1,1,2,2,3,3,3];
const uniqueArray=[...new Set(array)];
console.log(uniqueArray); //[1,2,3]
Permalink5) Truncate arrays
length is very popular property for getting the total numbers of elements in the array. But not many people realize we can use it as a setter too. Let me explain you with code.
let array=[1,2,3,4,5,6];
console.log(array); //[1,2,3,4,5,6]
console.log(array.length); //6
array.length=3 //deleted elements from index 3 to the actual length of array
console.log(array); //[1,2,3]
//we can also empty the array completely
array.length=0;
console.log(array); // []
Permalink6) Short circuit evaluation
&& or || can be used to quickly check for condtions and return results in a single line. It is a common pattern in React for conditional rendering. (&& is more popular than ||).
const componentShow = true;
const Component = () => <div>Our Content</div>;
//Component will be rendered
componentShow && <Component />;
const isLogIn = false;
const Login = () => <div>Login</div>;
//Component will not be rendered
isLogIn || <Login />;
Permalink7) Shuffle items in an Array
We can use sort() to randomly shuffle items in an array. It is very useful for browser games, when we want to randomize something. Have a glace at our code.
const numbers = [1, 2, 3, 4, 5, 6, 7, 8];
const numbersShuffle = numbers
.slice() //we use slice to copy an array
.sort(function () {
return Math.random() - 0.5;
});
console.log(numbersShuffle(numbrs)); //[2, 6, 4, 3, 1, 8, 7]
Permalink8) Remove false values from an Array
We can combine Boolean map method to quickly remove false values from an array. It is very useful when we expect null or other false values in an array and need to clean it.
const array = [1, 0, undefined, 6, 8, "", false, false];
console.log(array.filter(Boolean)); //[1,0,6,8]